Getting Started With Svelte: Introduction
Guide on how to get started with Svelte, including installation, component , state/props and rendering.
I've worked with Svelte on multiple projects and it's really great. It's easy to use and has lots of helpful features. The thing about Svelte is how fast it is and great developer experience. It uses a special way of making code that's smaller and faster than other frameworks. This will be a multi-part series on Svelte and its features. I will share my experience with Svelte while explaining how to start using it for production.
# What is Svelte?
Svelte is a next-generation web framework that enables developers to build highly performant web applications using a radically different approach. Like React and Vue.js , Svelte is component-based and is actually a compiler written in TypeScript that compiles your code at build time.
Unlike React, Svelte doesn’t use virtual DOM.The advantage of Svelte being a compiler is, it doesn’t use virtual DOM. In a nutshell, virtual DOM is a virtual representation of the DOM that's kept in memory and synced with the actual DOM. Libraries and frameworks like React and Vue.js use an algorithm called DOM diffing to compare the virtual DOMs and detect the necessary updates in the UI. Although algorithms for DOM diffing are efficient, they can be expensive to run.
Svelte, on the other hand, compiles code into optimized vanilla JavaScript, minimizing overhead at runtime. This approach speeds up applications and improves their performance. On top of that svelte is truly reactive. This means that the change in the variable will directly affect the UI. Svelte does this by generating code for each part of your program that updates the user interface directly, instead of comparing the current and previous versions of the user interface to see what changed. My most loved thing about Svelte is how it is minimal to write. Svelte needs a lot less code compared to other frameworks.
# Getting started with Svelte
To get started with Svelte, You can install Svelte using Node.js
on your machine. Once you make sure you have all the requirements install run this command to create a new svelte project with vite
.
pnpm create vite@latest svelte-demo -- --template svelte
This will create a new Svelte project in the svelte-demo folder. You can then start the development server using the pnpm dev
command. This will compile your code and start a local development server at http://localhost:5173 .
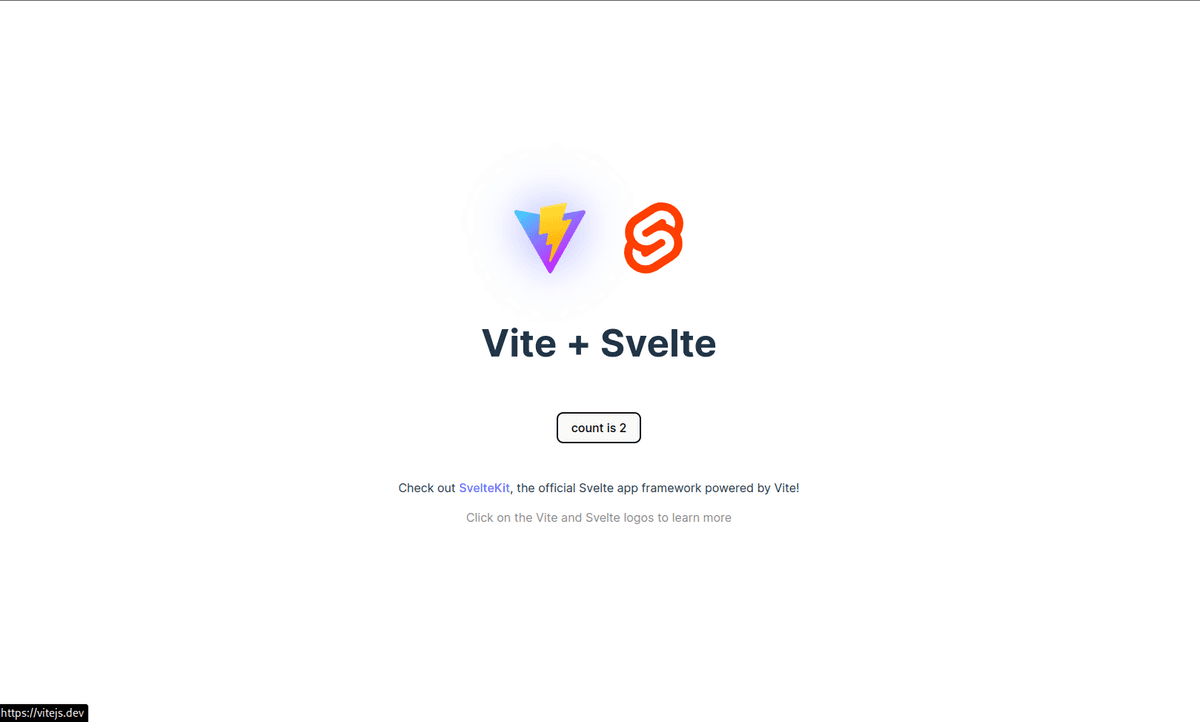
# Svelte components
A component is a self-contained atomic part of an application that may optionally reference other components to compose its output. A component can contain other components or be contained by another component, allowing for a hierarchical structure of components.
In other words, a component is an atomic part of the application. Examples of components include a form or an input element. In fact, the whole application itself can be a component. Svelte components contain all the necessary information to render a piece of the UI such as HTML for markup, JavaScript for behavior, and CSS for styling the UI.
In Svelte, an application is composed of one or more components. A component is a reusable, self-contained block of code that encapsulates HTML, CSS, and JavaScript that belong together. This code is written into a .svelte
file. In your created new project take a look at the App.svelte
file.
<script>
import svelteLogo from './assets/svelte.svg'
import viteLogo from '/vite.svg'
import Counter from './lib/Counter.svelte'
</script>
<main>
<!-- Regular HTML Tags -->
</main>
<style>
/* Regular CSS */
</style>
Take a look at the main.js
file in our project, we import the App.svelte
component to render it in our application. We also specify the target element where the component should be rendered. Here, we are rendering the component in an element with the ID of the app. This allows the component to be rendered in the browser when the application loads.
import './app.css'
import App from './App.svelte'
const app = new App({
target: document.getElementById('app'),
})
export default app
We can write an entire application by nesting components inside the App
component. Each component is defined in its own .svelte
file and can be imported into other components.
The App
component is typically the main component that holds all the other components. It's where we define the basic structure and layout of the application.
We can simplify the app by creating three components: Header
, Footer
, and Main
. Header
will display the logo and navigation, Footer
will include copyright details and links to social media, and Main
will show the main content of the application.
To add these components to the App
component, we simply import them and include them in the code.
<script>
import Header from './Header.svelte'
import Footer from './Footer.svelte'
import Main from './Main.svelte'
</script>
<Header />
<Main />
<Footer />
This code will show the Header, Main, and Footer parts inside the App part. By splitting the application into smaller parts, we can make the code easier to reuse, manage, and change.
# States and props in Svelte components
In Svelte, a component can have a state. A state is a variable that holds data used by the component. When the state changes, the component automatically updates the UI to show the new state.
To set a state, we use the let
keyword. Below is an example of a component that has a state:
<script>
let count = 0
function handleClick() {
count += 1
}
</script>
<button on:click={handleClick}>
Count: {count}
</button>
In this example, the count
variable is a container for a number. Every time the button is clicked, the handleClick
function is called and adds one to the count
container. The UI will then show the updated count
value automatically. States allow us to make UIs that automatically update as the user interacts with them.
# Props in Svelte Components
Svelte components can receive props, which are values that come from their parent component.
To pass a prop to a component, we use the props
attribute inside the component tag. The value of the attribute is an object with key-value pairs for each prop we want to pass. Here's an example:
<script>
export let name
</script>
<h1>
Hello, {name}!
</h1>
In this example, the name
prop is passed to the component using props
. The component uses the let
keyword to declare that it expects a name
prop. This allows the component to receive the name
prop and use it in the template.
When we use the component in another component, we pass the name
prop using the props
attribute:
<script>
import Greeting from './Greeting.svelte'
</script>
<Greeting name="John" />
In this example, we import the Greeting
component and pass it a name
prop with the value "John"
. The Greeting
component then displays the message "Hello, John!".
Props can also have default values. If a prop is not passed to the component, it will use the default value instead. Here's an example:
<script>
export let name = "Anonymous!"
</script>
<h1>
Hello {name}!
</h1>
In this example, the name
prop has a default value of "Hello, Anonymous!"
. If the component is used without passing a name
prop, it will display the default value.
<Greeting />
Output!
<h1>
Hello, world!
</h1>
Inside the curly braces, we can put any JavaScript we want. For example, we can modify a string to be more shouty by using the .toUpperCase() function. Here's an example:
<script>
export let name = "Anonymous!"
</script>
<h1>
Hello {name.toUpperCase()}!
</h1>
You can also create a read only props with export const name = "Admin"
We can also use the spread operator (...)
to pass all the properties of an object or an array as separate props. Here's an example of passing all the properties of an object as separate props:
<script>
let person = {
name: 'John',
age: 30,
location: 'New York',
}
</script>
<!-- Pass all the properties of the person object as separate props -->
<Person {...person} />
In this example, we're using the spread operator to pass all the properties of the person
object as separate props to the Person
component.
# Reactivity in Svelte
In Svelte, you can listen for changes in the component state and update other variables. This is done using reactive assignments. A reactive assignment is a special assignment that updates a variable whenever another variable changes. Here's an example:
<script>
let count = 0
function increment() {
count += 1;
}
$: doubled = count * 2
</script>
<button on:click={increment}>
Count: {count}, Doubled: {doubled}
</button>
In this example, we have a variable called count
. We use a reactive assignment to create a new variable called doubled
. This variable is equal to count * 2
. Whenever count
changes, doubled
updates to reflect the new value.
Reactive assignments are created using $:
syntax. When the variables depend on change, the expression after $:
will be re-evaluated.
You can just reassign a variable to trigger UI update as well. For example,
<script>
let count = 0
function increment() {
count += 1;
}
function reset(){
// trigger a UI update
count = 0
}
$: doubled = count * 2
</script>
<button on:click={increment}>
Count: {count}, Doubled: {doubled}
</button>
<button on:click={reset}>
Reset
</button>
Checkout more reactive example here .
# Updating arrays in Svelte
In Svelte, you can change arrays using methods like push
, pop
, shift
, unshift
, splice
, and sort
.
<script>
let todoList = ['Finish Svelte tutorial', 'Start Svelte project']
function addTodo() {
todoList = [...todoList, 'New Todo']
}
function removeTodo() {
todoList.pop()
todoList = todoList
}
</script>
<h2>Todo List</h2>
<ul>
{#each todoList as todo}
<li>{todo}</li>
{/each}
</ul>
<button on:click={addTodo}>Add Todo</button>
<button on:click={removeTodo}>Remove Todo</button>
We have a list called todoList
with two items. We add a new item to the end of the list using the spread operator. We remove the last item from the list using the pop
method.
# Conditional Rendering in Svelte
Svelte has its own logic blocks for conditionally rendering contents. We can use the if
block to conditionally render content in Svelte. Here's an example:
<script>
let isLoggedIn = false
</script>
{#if isLoggedIn}
<p>Welcome back!</p>
{:else}
<p>Please log in.</p>
{/if}
We have a variable named isLoggedIn
. We use the if
block to display content depending on the value of isLoggedIn
.
We can also use the else if
block to add more conditions:
<script>
let isLoggedIn = false
let isAdmin = false
</script>
{#if isLoggedIn}
<p>Welcome back!</p>
{:else if isAdmin}
<p>Welcome back, admin!</p>
{:else}
<p>Please log in.</p>
{/if}
We can also use the each block to loop through arrays for rendering multiple contents
<script>
let todoList = ['Finish Svelte tutorial', 'Start Svelte project']
</script>
<h2>Todo List</h2>
<ul>
{#each todoList as todo}
<li>{todo}</li>
{/each}
</ul>
In this example, we have an array called todoList
with two items. We use the each
block to loop through the array and render an <li>
element for each item.
You can check out more on logic blocks at the official documentation .
Rendering content with promise in svelte,
{#await promise}
<!-- promise is pending -->
<p>waiting for the promise to resolve...</p>
{:then value}
<!-- promise was fulfilled -->
<p>The value is {value}</p>
{/await}
I hope the introduction to Svelte has been helpful to you. If you need more information, feel free to reach out. I'll be posting more articles to help you learn Svelte and share my experiences. Additionally, if you're just starting out with Svelte, I highly recommend checking out the quick-start tutorial for beginners.