Var.Camp 2.0 Supercharging your Javascript development with Typescript
My brief catch up at var.camp 2.0.
I recently participated in var.camp 2.0, an online camp where people from all over the world come together to share their experiences and knowledge on various topics.
One of the things I loved most about var.camp was the opportunity to listen to and learn from people's experiences. Each participant had their own unique story to tell and their own insights to share.
From learning how to code in new programming languages to understanding different perspectives on personal growth, the variety of topics covered at var.camp was awe-inspiring. I was particularly drawn to Typescript and decided to talk about what I have learned. This article is about briefing what I shared at var.camp 2.0.
# Javascript is free!
In JavaScript, you can re-declare variables and objects as much as you want. This means that you can change the value of a variable or object multiple times throughout your code without any errors or issues. Of course, as with any programming language, it is essential to use caution when re-declaring variables and objects. If you are not careful, you can accidentally overwrite essential data or create conflicts that cause your code to break.
function countCharacter(value){
return value.length
}
var name = "Danger"
countCharacter(name) // 5
var name = 1
countCharacter(name) // ?
var name = false
countCharacter(name) // ?
When it comes to documentation, it is very hard to maintain in the future, especially in unfamiliar code bases.
By using Typescript, we have added more information and clarity to our code, making it easier to understand and maintain over time. This is just one example of how Typescript's type system can improve code documentation and maintenance.
interface Address {
street: string;
city: string;
state: string;
zipCode: string;
}
interface User {
name: string;
email: string;
password: string;
address: Address;
}
function createUser(name: string, email: string, password: string, address: Address): User {
return {
name,
email,
password,
address: address
};
}
const address = {
street: "123 Main St",
city: "Anytown",
state: "CA",
zipCode: "12345",
};
const newUser = createUser("John Doe", "john@example.com", "secret", address);
# Stronger developer toolings
TypeScript's strong typing system can greatly improve a developer's productivity by providing them with more powerful and intelligent developer tooling.
For example, with TypeScript, code editors and IDEs can provide more advanced code completion and error detection features. The editor can suggest function names, variable types, and method signatures as the developer is typing, reducing the time it takes to write the correct code. It can also immediately flag errors and potential issues, helping the developer catch mistakes early on and saving time in the long run.
In addition, TypeScript can help reduce the need for manual testing and debugging by catching errors earlier in the development process. This can save a significant amount of time and effort, allowing developers to focus on building and improving the codebase instead of fixing bugs.
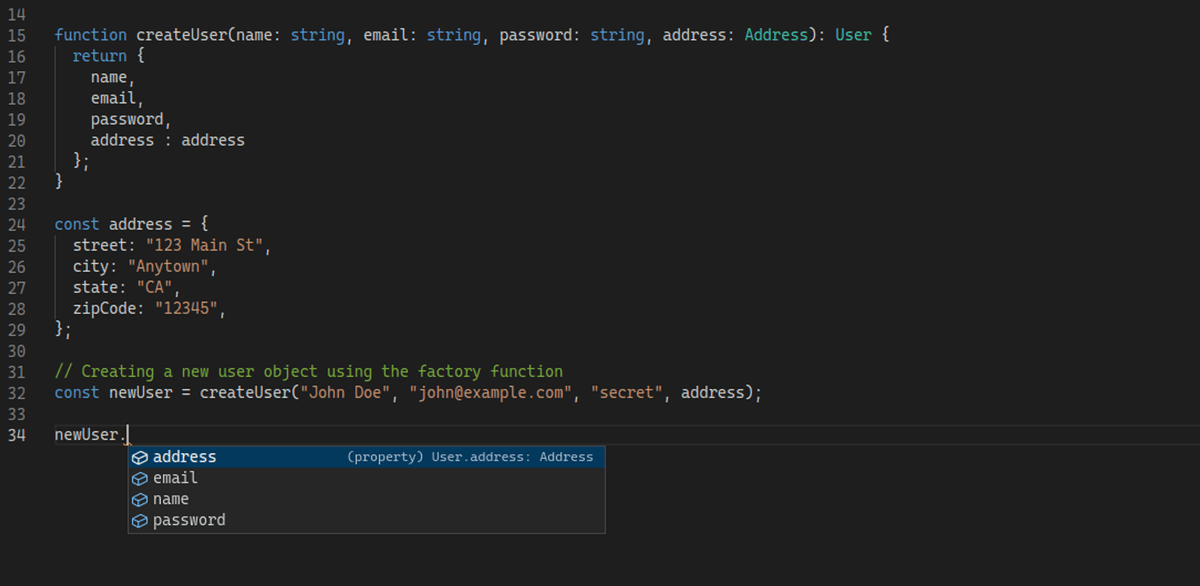
# Adapt incrementally
I discussed how you can migrate your Javascript code base incrementally to Typescript. In general, every valid JavaScript code is also a valid TypeScript code. This is because TypeScript is designed to be a superset of JavaScript, meaning that any valid JavaScript code is also valid TypeScript code.
The reason for this is that TypeScript extends JavaScript by adding additional syntax and features such as strong typing, interfaces, and classes. However, TypeScript does not remove or change any of the existing JavaScript syntax, so any valid JavaScript code can still be used in TypeScript.
This compatibility with JavaScript makes it easy for developers to incrementally adopt TypeScript in their existing JavaScript codebases. You can start by adding TypeScript to new files or gradually converting existing JavaScript code to TypeScript, without having to rewrite their entire codebase from scratch.
If you have an existing JavaScript code base and want to start using TypeScript. Begin by writing new code in TypeScript, while keeping your existing JavaScript code as is.
This way, you can use TypeScript's strong typing system for new code, while still using your existing JavaScript code-base. You can incrementally adopt TypeScript in a way that minimizes disruption to your existing code and team workflows.
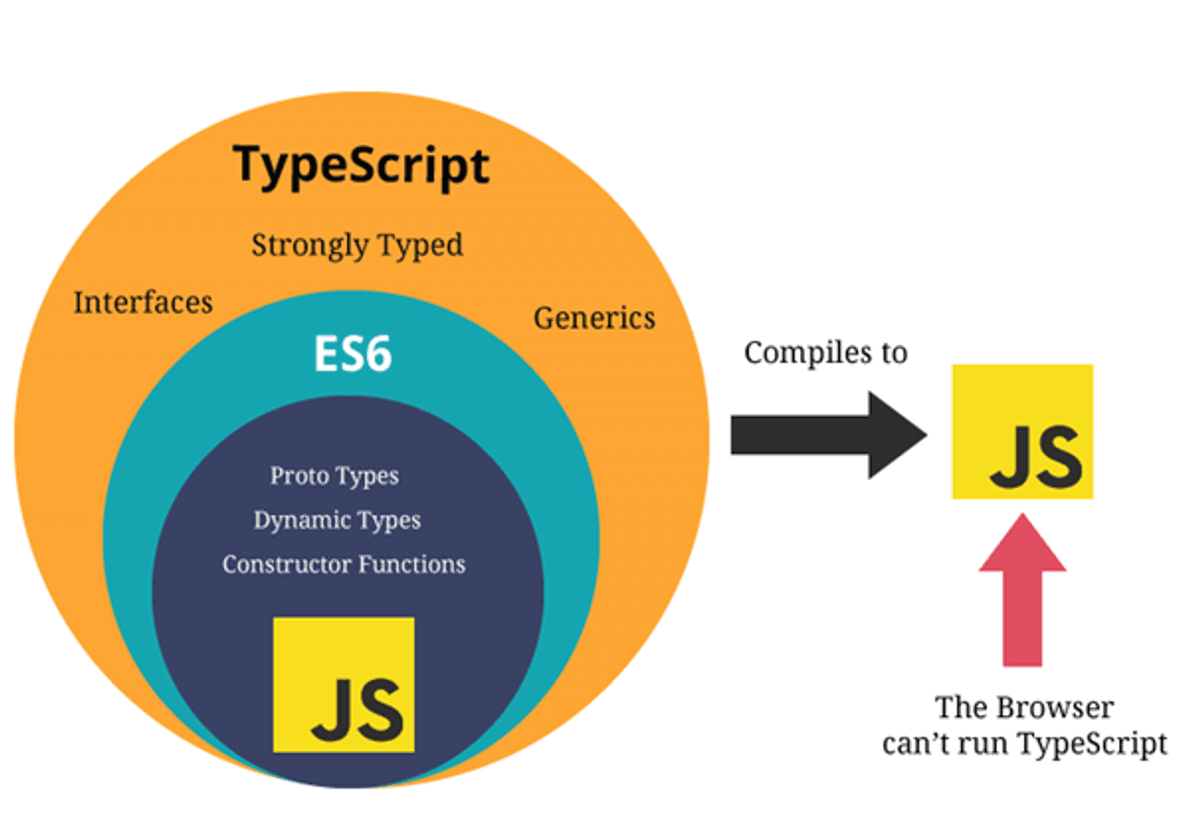
# Some basic Typescript
Then I came up with some of the basic data types in Typescript and how you can effectively use them. In addition to the basic data types, I discussed how TypeScript provides several "everyday" types that are commonly used in programming.
For example, union type which allows developers to define a variable or parameter that can accept multiple types. This is useful when a function or method can accept different types of inputs. Another type is the top type, which represents a value that can be any type.
This is useful when you need to work with values that have unknown or dynamic types. Also how TypeScript provides support for generics, which allows developers to define functions and classes that can work with multiple types.
function typePredicate(input: WideType): input is NarrowType;
function isNumberOrString(value: unknown) : value is number | string {
return ['number', 'string'].includes(typeof value);
}
function logValueIfExists(value: number | string | null | undefined) {
if (isNumberOrString(value)) {
// 😍 Type of value: number | string
console.log(value.toString());
} else {
// Type of value: null | undefined
console.log("value does not exist:", value);
}
}
logValueIfExists(null)
I discussed how to use declaration files and how they provide TypeScript with the necessary information to properly type-check code that uses the library or module, which is especially useful when working with JavaScript libraries that do not have built-in TypeScript support.
// styles.d.ts
declare module "*.module.css" {
const styles: { [i: string]: string };
export default styles;
}
// component.ts
import styles from "./styles.module.css";
styles.anyClassName; // Type: string
// globals.d.ts
declare const version: string;
// version.ts
export function logVersion() {
console.log(`Version: ${version}`); // Ok
}
# Definitely typed
After that, I discussed on DefinitelyTyped, which is a community-driven project that provides high-quality TypeScript declaration files for popular JavaScript libraries and modules. Discussed how these declaration files provide TypeScript with the necessary information to properly type-check code that uses the external code, ensuring that the code is more reliable and easier to maintain.
# IDE features
Then how TypeScript provides several IDE features that can significantly improve developer productivity and make writing code easier and more efficient. Such as code completion, which is available in most modern IDEs. Code completion provides suggestions for completing code snippets as you type, saving time and reducing errors.
Talked about how you can use features like go-to-definition, find-all-references, and refactoring, which can make it easier to navigate large codebases and make changes to the code without introducing errors.
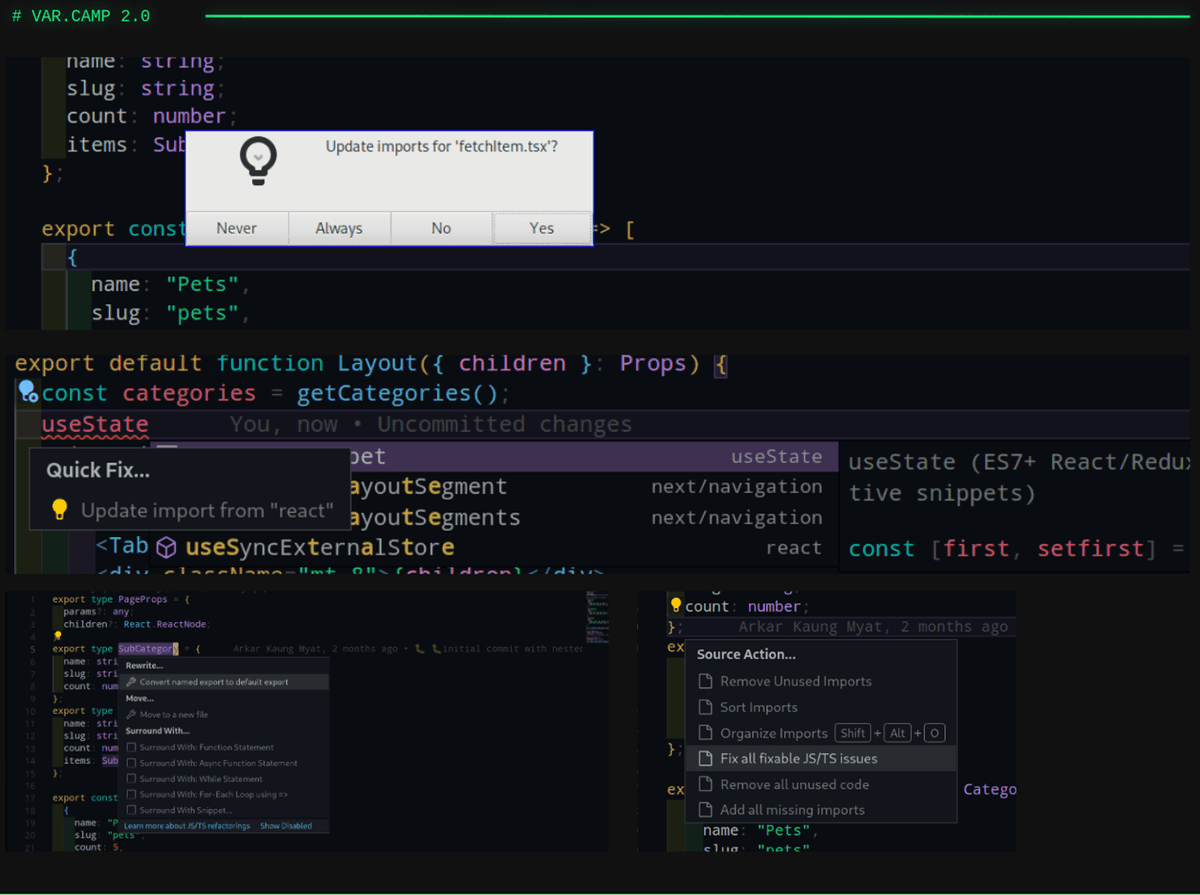